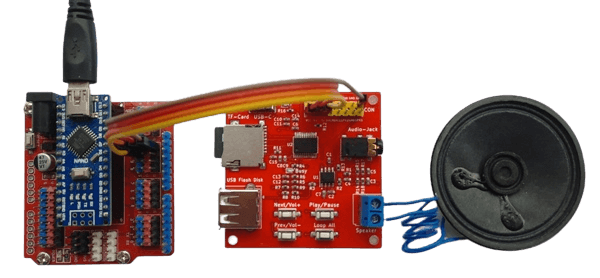
- Arduino Nano D10 接 Mp3 Board Tx
- Arduino Nano D11 接 Mp3 Board Rx
- Arduino Nano Vin 接 Mp3 Board Vcc
- Arduino Nano GND 接 Mp3 Board GND
#include “SoftwareSerial.h”
SoftwareSerial mySerial(10, 11);
# define Start 0x7E
# define Ver 0xFF
# define Length 0x06
# define End 0xEF
# define Ack 0x00 // 0x01: ACK, 0x00: no ACK
void setup() {
mySerial.begin (9600); // Baudrate: 9600bps
delay(1000);
setVolume(25); // 設定音量 : 0~30
playNum(1); // 指定曲目
}
void loop() {
// put your main code here, to run repeatedly:
}
void playNum(int num) { //指定曲目
execute_CMD(0x03, 0, num); // Set the volume (0x00~0x30)
delay(1000);
}
void setVolume(int volume) { // 設定音量
execute_CMD(0x06, 0, volume); // Set the volume (0x00~0x30)
delay(1000);
}
// 執行命令及傳入參數
void execute_CMD(byte CMD, byte Par1, byte Par2) {
// 計算checksum (2 bytes)
word checksum = -(Ver + Length + CMD + Ack + Par1 + Par2);
// 建立命令列
byte Command_line[10] = { Start, Ver, Length, CMD, Ack,
Par1, Par2, highByte(checksum), lowByte(checksum), End};
// 送出命令列 to Gheng Mp3 Board
for (byte i=0; i<10; i++){
mySerial.write( Command_line[i]);
}
}
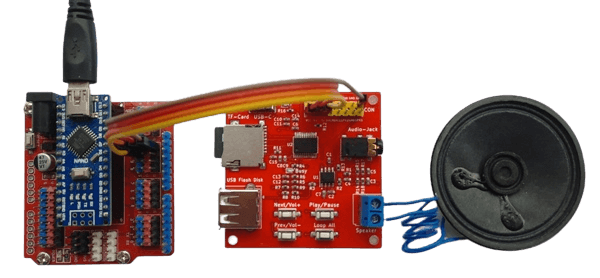
- Arduino Nano D10 接 Mp3 Board Tx
- Arduino Nano D11 接 Mp3 Board Rx
- Arduino Nano Vin 接 Mp3 Board Vcc
- Arduino Nano GND 接 Mp3 Board GND
使用 Arduino 函式庫DFRobotDFPlayerMini.h
#include “Arduino.h”
#include “SoftwareSerial.h”
#include “DFRobotDFPlayerMini.h”
SoftwareSerial mySoftwareSerial(10, 11); // RX, TX
DFRobotDFPlayerMini myDFPlayer;
void setup()
{
mySoftwareSerial.begin(9600);
Serial.begin(115200);
Serial.println();
Serial.println(F(“Gheng-MP3 Board Demo play”));
if (!myDFPlayer.begin(mySoftwareSerial)) { //Use softwareSerial to communicate with mp3.
Serial.println(F(“Unable to begin:”));
Serial.println(F(“1.Please recheck the connection!”));
Serial.println(F(“2.Please insert the TF card!”));
while(true);
}
Serial.println(F(“Gheng-MP3 online.”));
//—-Set different EQ—-
myDFPlayer.EQ(DFPLAYER_EQ_NORMAL);
// myDFPlayer.EQ(DFPLAYER_EQ_POP);
// myDFPlayer.EQ(DFPLAYER_EQ_ROCK);
// myDFPlayer.EQ(DFPLAYER_EQ_JAZZ);
// myDFPlayer.EQ(DFPLAYER_EQ_CLASSIC);
// myDFPlayer.EQ(DFPLAYER_EQ_BASS);
myDFPlayer.volume(20); //Set volume value. From 0 to 30
myDFPlayer.play(1); //Play the 1st mp3
}
void loop() {
// put your main code here, to run repeatedly:
}

8051: Nuvoton MS51FB9AE
#include “MS51_16K.H”
void delay_104us(); // Delay 104us for Baud Rate 9600bps, T=1/9600 = 104.167us
void send_byte(unsigned char byte_data);
void send_CMD(unsigned int CMD, parameter);
// MP3 Command data frame format:
// 0 1 2 3 4 5 6 7 8 9-byte
//Start, VER, Length, Command, Chip_ACK, Data_H, Data_L, Checksum_H, Checksum_L, End
static unsigned int CMD_Byte[10]=
{0x7E, 0xFF, 0x06, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0xEF};
sbit Serial_TX = P0^6;
// sbit Serial_RX = P0^7;
#define Reset_Chip 0x0C // Reset Mp3 chip
#define SetVolume 0x06 // 指定音量
#define SetNumber 0x03 // 指定曲目
void main (void)
{
P06_QUASI_MODE; // Setting UART Tx as Quasi mode
// P07_INPUT_MODE; // Setting UART Rx as input mode
send_CMD(Reset_Chip, 0x19); // Reset Mp3 chip
Timer0_Delay(24000000,840,3175); // 延遲 4 SEC = 840
send_CMD(SetVolume, 0x19); // 指定音量 0 ~ 30
send_CMD(SetNumber, 0x01); // 指定曲目
while(1);
}
void send_CMD(unsigned int CMD, parameter)
{
int checksum = 0;
send_byte(CMD_Byte[0]); // 0x7E ~ Start bit
send_byte(CMD_Byte[1]); // 0xFF ~ Ver
send_byte(CMD_Byte[2]); // 0x06 ~ Byte Length
CMD_Byte[3] = CMD;
send_byte(CMD_Byte[3]); // Command
send_byte(CMD_Byte[4]); // 01: ACK, 00: no ACK
CMD_Byte[5]= (parameter & 0xFF00)>> 8; // High byte for parameter
CMD_Byte[6]= parameter & 0x00FF; // Low byte for parameter
send_byte(CMD_Byte[5]); // High byte for parameter
send_byte(CMD_Byte[6]); // Low byte for parameter
checksum = -(CMD_Byte[1] + CMD_Byte[2] + CMD_Byte[3] + CMD_Byte[4] + CMD_Byte[5] + CMD_Byte[6]) & 0xFFFF;
CMD_Byte[7] = (checksum & 0xFF00) >> 8;
CMD_Byte[8] = checksum & 0x00FF;
send_byte(CMD_Byte[7]); // High byte for checksum
send_byte(CMD_Byte[8]); // Low byte for checksum
send_byte(CMD_Byte[9]); // 0xEF ~ Stop bit
}
void send_byte(unsigned char byte_data)
{
unsigned char i;
Serial_TX = 0; // UART Start-bit
delay_104us(); // 等待 104us,維持資料位元狀態
for(i=0;i<8;i++)
{
Serial_TX = byte_data & 0x01; // 設定輸出為最低位元,表示傳送資料位元
delay_104us(); // 等待 104us,維持資料位元狀態
byte_data = byte_data >> 1; // 資料位元向右移動一位,準備傳送下一個位元
}
Serial_TX = 1; // UART Stop-bit
delay_104us(); // 等待 104us,維持資料位元狀態
}
void delay_104us()
{
unsigned char i;
for (i = 0; i < 110; i++) {
_nop_();
_nop_();
_nop_();
_nop_();
_nop_();
_nop_();
_nop_();
_nop_();
}
}